#graphics#shader#hlsl#unity#csharp#experiment#solo
How to: Make a 2D Silhouette of the Player, Unity (Part 2)
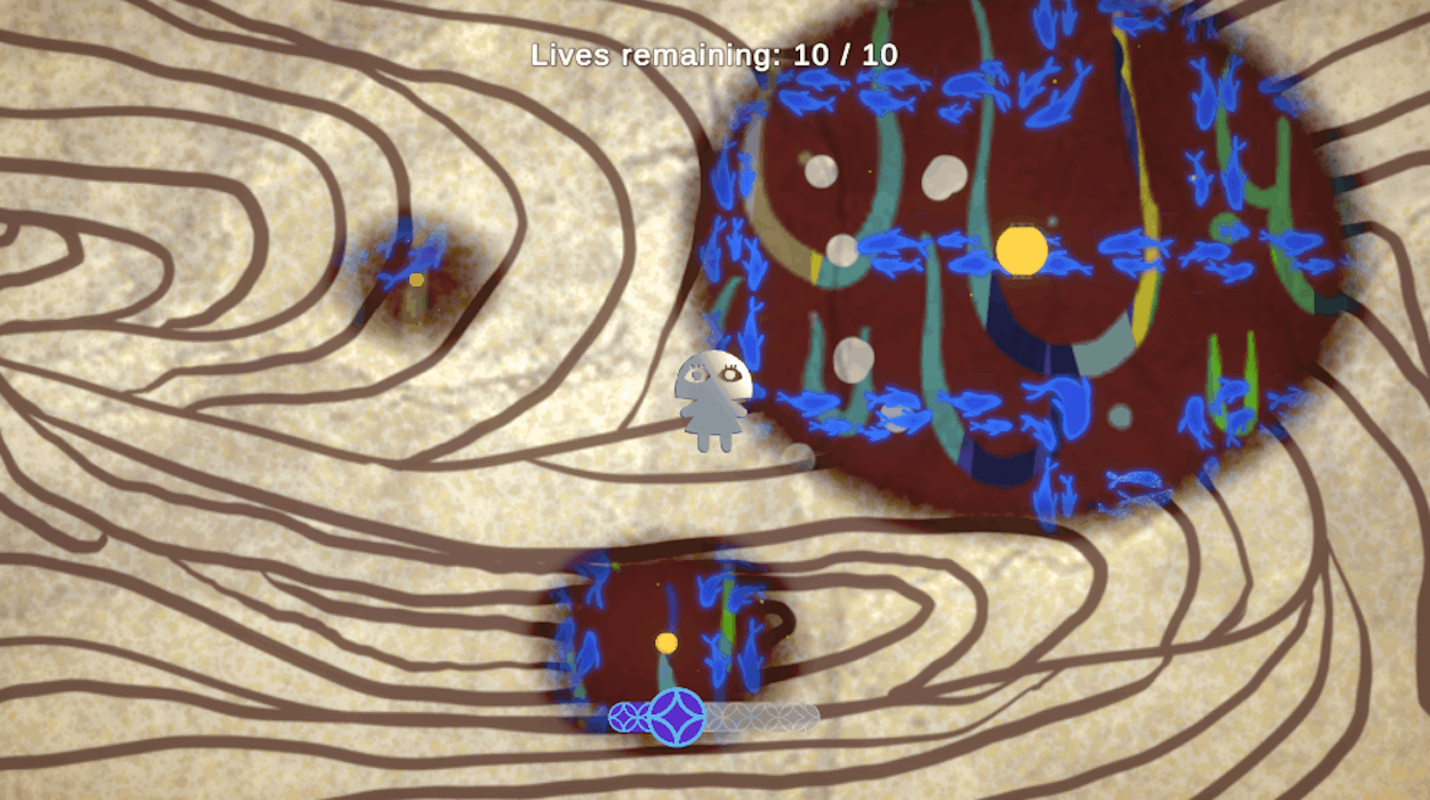
If you recall from the previous post, Luminth was able to render lights , illuminating the maze. But what about the player?
A good way to show where the player is in the mist of clouds is with a silhouette. This will require a copying of the player sprite on top of the clouds texture.
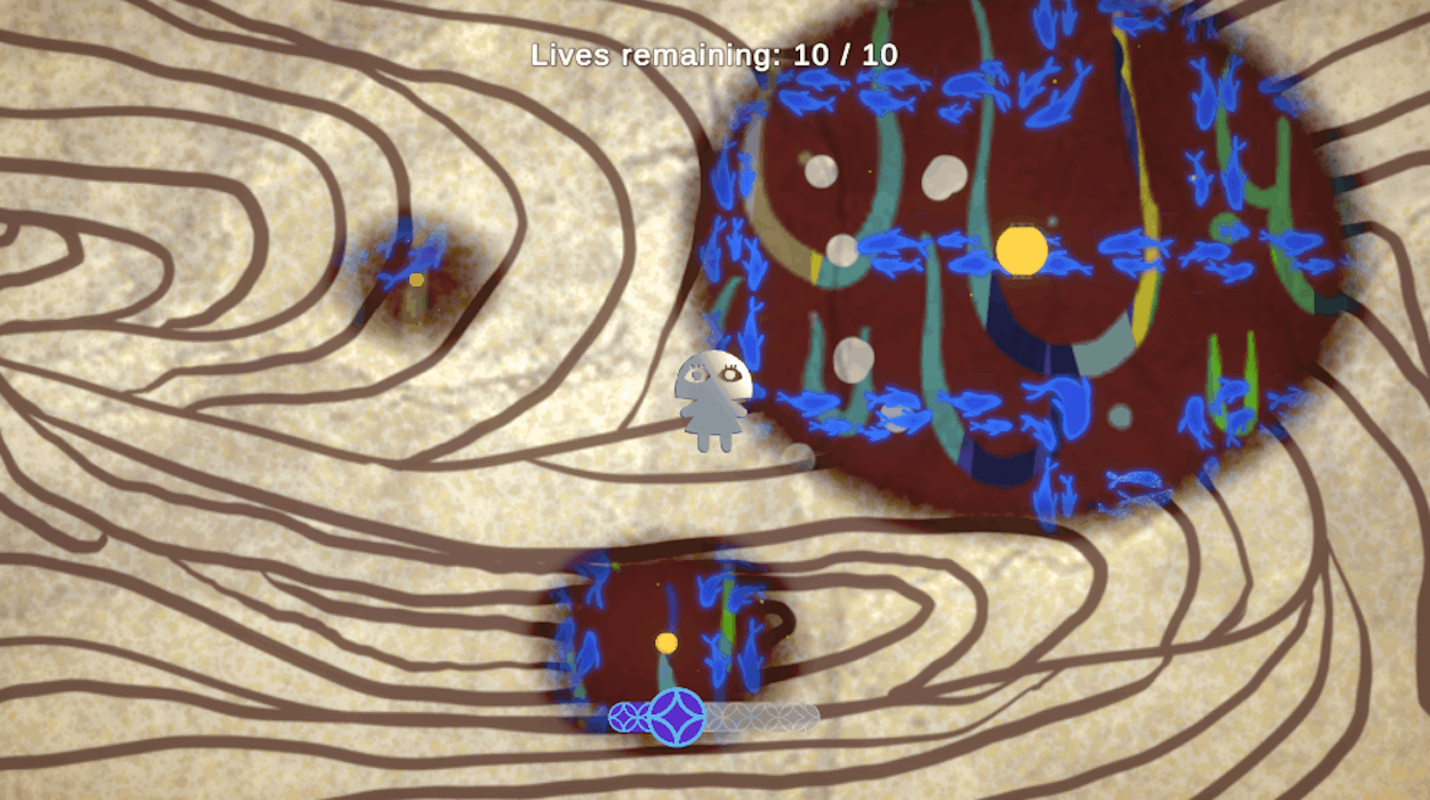
1. Define a player box, the position and space the player takes in the texture
v2f vert(appData v) {
...
float2 playerOrigin = _PlayerData.xy;
float2 playerRelativeScale = _PlayerData.zw;
o.playerBox = playerRelativeScale * (v.uv - playerOrigin);
}
This requires a _PlayerData property, that should be passed using a C# script
2. Define the function to draw a full silhouette copy of the player
fixed4 renderSilhouette(fixed4 col, float2 playerBox) {
// Get the Player Texture Color
fixed4 player = tex2D(_PlayerSprite, playerBox);
// Fill the sprite transparent areas with the underlying background
// This will render the silhouette player at all times
// if player.a = 0, draw the clouds
// if player.a = 1, draw the player
return lerp(col, _SilhouetteColor.rgba, player.a);
}
Taking the player's sprite, and outputting a clean, gray-colored version of it
3. Draw the silhouette, before the alpha blending of the cloud holes
fixed4 frag(v2f i) : SV_Target {
fixed4 col = tex2D(_CloudTex, i.uv);
// Within the bounds of the player texture at it's position
if(i.playerBox.x > 0 && i.playerBox.x < 1) {
if(i.playerBox.y > 0 && i.playerBox.y < 1) {
col = renderSilhouette(col, i.playerBox);
}
}
col = renderCloudHoles(col, i.uv);
return col;
}
It was a lot more simpler than initially anticipated! I believe that there should be a better way to write out o.playerBox, but this implementation 100% works.
Source Code: Clouds.shader Source Code: Clouds.csThank you for reading! Check out the game that used this, or the project details about it here!
Luminth Details